Users guide¶
Installation¶
There is no special installation required. It can be downloaded from https://github.com/vondrejc/FFTHomPy.git or using Git by:
git clone https://github.com/vondrejc/FFTHomPy.git
The code is implemented in python and supports versions 2 and 3.
Running the program¶
Command line usage:
$ python main.py examples/scalar/scalar_2d.py
or only as:
$ ./main.py examples/scalar/scalar_2d.py
where examples/scalar/scalar_2d.py
is an input file for FFTHomPY.
Definition of input file¶
Input file for FFTHomPy consists of material definition and problem definition.
Material definition¶
Material coefficients can be defined as matrix-inclusion composites or grid-based composites.
Matrix-inclusions composites¶
In this case, material is expressed at points of periodic cell
as
where functions describe inclusion topologies located at
with material coefficients
.
An example of material coefficients is named
'square'
import numpy as np
materials = {'square': {'Y': np.ones(dim),
'inclusions': ['square', 'otherwise'],
'positions': [np.zeros(dim), ''],
'params': [0.6*np.ones(dim), ''],
'vals': [11*np.eye(dim), 1.*np.eye(dim)]}}
and the used keywords have following meanings:
'Y'
:numpy.array
of shape(dim,)
describes the size of periodic cellin dimension
dim
'inclusions'
: list of inclusionsof following types
'square'
,'circle'
, and'otherwise'
in two-dimensional settings'cube'
,'ball'
, and'otherwise'
in two-dimensional settings
'positions'
: list of positionscorresponding to individual inclusions
- the position corresponds to center of gravity with respect to coordinate system; the inclusion
'otherwise'
has no position because it represents the area in periodic cell omitted by inclusions
'params'
: list of parameters determining the inclusions
- for
'square'
and'cube'
, it corresponds to sizes of individual sides- for
'circle'
and'ball'
, it corresponds to diameter
'vals'
: list of material coefficients for individual inclusions; coefficients are represented asnumpy.array
of shape corresponding to physical problem according to problem definition; for scalar elliptic problem, the shape is(dim, dim)
while for linearized elasticity the shape is(D, D)
whereD = dim*(dim+1)/2
.
Grid-based composites¶
Contrary to Matrix-inclusions composites, grid-based composites are defined on grid points:
for some number of points and the size
of periodic cell
; examples for odd and even grids are depicted in following figure
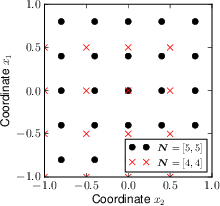
for periodic cell with the cell size
.
The material is then approximated with the following formula
(1)
where function is taken either by
(2)
leading to piece-wise constant approximation of material coefficients, or by
(3)
leading to piece-wise bilinear approximation of material coefficients.
In comparison to Matrix-inclusions composites, the material coefficients definition
materials.update({'square_Ga': {'Y': np.ones(dim),
'inclusions': ['square', 'otherwise'],
'positions': [np.zeros(dim), ''],
'params': [0.6*np.ones(dim), ''],
'vals': [11*np.eye(dim), 1.*np.eye(dim)],
'order': 0,
'P': 5*np.array(dim)}})
Problem definition¶
Here, the example of problem description is stated:
problems = [{'name': 'prob1',
'physics': 'scalar',
'material': 'square',
'solve': {'kind': 'GaNi',
'N': N,
'primaldual': ['primal', 'dual']},
'postprocess': [{'kind': 'GaNi'},
{'kind': 'Ga',
'order': None},
{'kind': 'Ga',
'order': 0,
'P': N},
{'kind': 'Ga',
'order': 1,
'P': 27*N}],
'solver': {'kind': 'CG',
'tol': 1e-6,
'maxiter': 1e3}}]
- The individual keywords are explained:
'name'
: the name of a problem'physics'
: defines the physical problem that is solved; following alternatives are implemented:'scalar'
: scalar linear elliptic problem (diffusion, stationary heat transfer, or electric conductivity)'elasticity'
: linearized elasticity (small strain)
'material'
: keyword refering to dictionarymaterials
or directly dictionary defining the material coefficients'solve'
: defines the problem discretization, the way how to solve minizers (corrector functions)'kind'
: is either'Ga'
(Galerkin approximation) or'GaNi'
(Galerkin approximation with numerical integration); it thus corresponds to the discretizaiton way'N'
: is anumpy.array
defining the approximation order of trigonometric polynomials; the higher the value is, the better approximation is provided'primaldual'
: determine if primal, dual, or both formulations are calculated
'solver'
: defines the linear solver and relating parameters'kind'
: linear solver one of'CG'
for Conjugate gradients,'BiCG'
for Biconjugate gradients,'richardson'
for Richardson’s iterative solution,'scipy_cg'
forscipy.sparse.linalg.cg
, and'scipy_bicg'
forscipy.sparse.linalg.bicg
,'tol'
: the required tolerance (float) for the convergence of linear solver'maxit'
: the maximal number of iterations
'postprocess'
: defines the way for calculating homogenized material coefficients from minimizers that are obtained with a way defined in'solver'
'kind'
: is either'Ga'
(Galerkin approximation) or'GaNi'
(Galerkin approximation with numerical integration); it thus corresponds to the discretizaiton way'order'
: applicable only for'Ga'
, it defines approximation order according to (2) or (3)'P'
: applicable only for'Ga'
, thisnumpy.array
of shape(dim,)
describes the resolution of approximation in (1)